Remove characters from strings using Regex in Power Apps
This tutorial will show you how to remove characters from strings using Regex in Power Apps. This can also be applied to a text input, label, or anything that is string-based.
I’ve seen many tutorials out there that show you how to detect characters using Regex, and then have some kind of “Special characters are not allowed” message, and I thought to myself:
There must be a better way! (“There is always a better way”)
Wouldn’t it just be easier if we stop the user from entering those characters by removing any that they have typed?
In some cases we may need to ensure folder structures are valid or to ensure that data passed into other systems does not contain any breaking characters – relying on our user to clean up their data leaves it open to potential issues and data clean-up nightmares!
There are many ways for us to implement this, and I will give you one here that can then be modified or expanded to your own needs.
(EDIT: UPDATE, due to changes to how Distinct and Split output in the newest editor versions, these formulas have been updated – read more here)
Let’s start with a simple example and we can expand on that as we go.
Reference one label from another label
Firstly, we create two labels.
Label1’s Text is “qwerty!@£$%^&*()<>?:,./;uiop”
Then we reference Label1 from Label2 by setting Label2’s Text property to: Label1.Text

Split your string into an array of characters
Now we want to evaluate each individual character separately, so the way to get at each character is to use the Split() function but instead of splitting by a particular character, we are going to split by no character (“”) which will split it on every character. This will give us a table of data containing a row for each character, which we can then iterate against.
Split(Label1.Text, “”)
Reconstitute your array into a string
Ok, so now we are going to recombine those letters back into a string by using the Concat() function. This is so that we can reach the next step of evaluating each character. If we highlight the split in our formula we can see the table (array) of characters.

Remove characters from strings by adding some magical Regex Wizard-sauce
Now we add the magic. I’m not going to give a whole primer on how the heck Regex (Regular Expressions) work, but I will give a brief explanation. If we use the IsMatch function, we can check against a Regex string to see if it matches or not and then either do something or else do something else – Regex strings are basically a formulated way of determining which characters and in which sequence and number they are allowed. This can be used to validate and change strings based on the rules set out by the Regex string.
In this example, We start by defining a group with an open round bracket, then we define a character section using a square bracket, then I am only going to allow Characters A-Z (Uppercase letters) and Characters a-z (lowercase letters) and Characters 0-9 (all numbers) and the ‘space’ character, then we close that section with a close square bracket and close the group with a close round bracket.
([A-Za-z0-9 ])
Which, in our formula, becomes:
Concat( Split(Label1.Text, "" ), If( IsMatch(Value, "([A-Za-z0-9 ])" ), Value ) )

ok now let’s add some spaces throughout to ensure it is actually keeping the spaces!

Remove characters from strings automatically after being typed
If we want to remove characters from strings but have it done automatically, there are a few ways to do this. To implement the next improvement (without running into any circular reference issues) we need to create a variable that can be manipulated, so I have inserted one Text Input, and on its OnChange I have put the following:
Set(gblTextRegex, Self.Text)
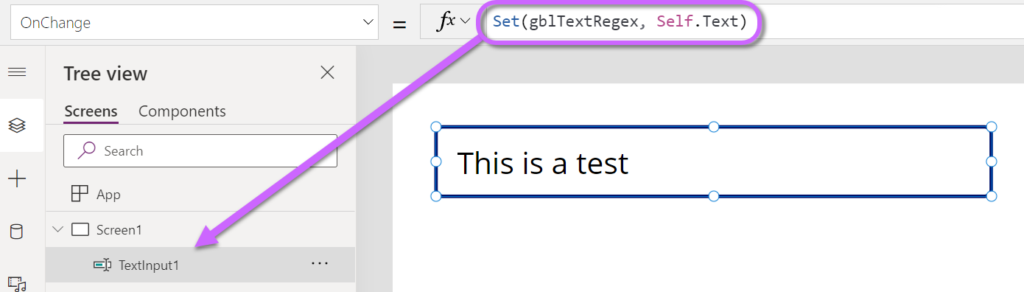
This means that every time the user finishes typing into the Text input, it will set that variable to whatever Text is inside that Text Input control.
Now in order to have it change we simply need to change the Default of that control to use that Regex formula from above but instead we are going to reference the Variable instead of the Text property.
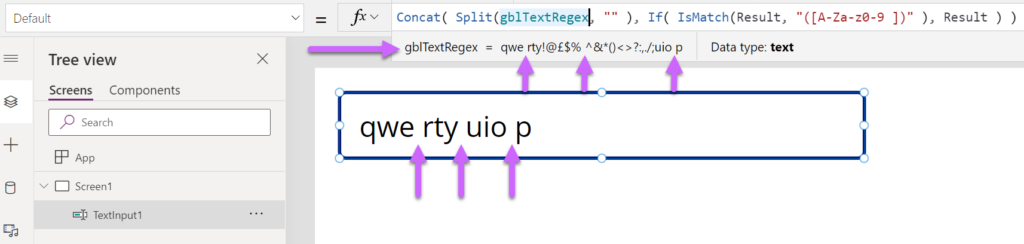
This will mean that when the user finishes typing and clicks into another field then it will immediately change the text value to remove the characters from the string. In the above example I just retyped the exact same string we used in the previous example with spaces in between some of the characters, and as soon as I clicked out of the Text Input it removed the invalid characters.
Replace special characters with something else
We already have all the work done, we just need to add an ‘else’ condition inside our ‘If’ condition. I will replace all of the special characters here with a * character:
Concat( Split(gblTextRegex, "" ), If( IsMatch(Value, "([A-Za-z0-9 ])" ), Value, "*" ) )

Only show special characters
There are a few ways to do this by moving around where the logic happens but I’m going to show how to do with by modifying the Regex string.
You can use ^ to indicate NOT , so the string becomes:
([^A-Za-z0-9 ])
and then the code becomes:
Concat( Split(gblTextRegex, "" ), If( IsMatch(Value, "([^A-Za-z0-9 ])" ), Value) )
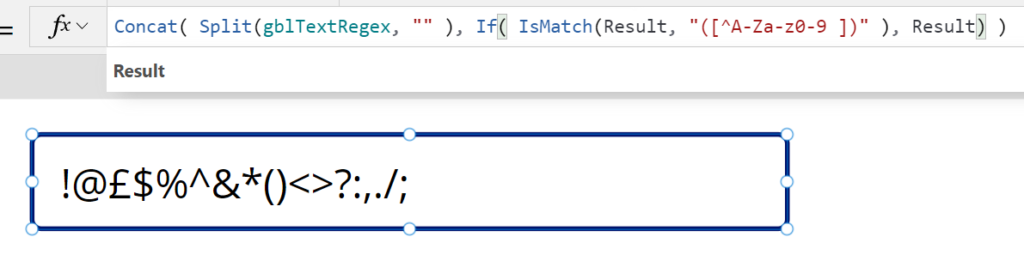
Add ‘allowed’ characters
We can modify the string to allow certain characters through, i.e. if we want the @ symbol to be allowed through we can add @ to the regex string. If we want to create a way to remove characters from string but also to ensure email addresses are never allowed to have spaces we can also remove the space from our string and add an allowed period symbol:
Concat( Split(gblTextRegex, "" ), If( IsMatch(Value, "([A-Za-z0-9@.])" ), Value) )

Change the text AS YOU TYPE
Ok, so this is where it gets interesting – we will be adding a timer, set to repeat and length 500ms.
Now we remove the OnChange value of the Text Input and change the timer’s OnTimerStart to:
Set(gblTextRegex, Concat( Split(TextInput1.Text, "" ), If( IsMatch(Value, "([A-Za-z0-9 ])" ), Value))); Reset(TextInput1)
We also change the Default of the Text Input to the variable gblTextRegex:


So, now we can type all sorts of special characters and this GIF below demonstrates how this is handled using our formula:
*Credit to Matthew Devaney for advising me on the need for the Reset() function to fix the random error that had popped up 😹

Replace (Or Remove) two characters matching a Regex string
We can also look at replacing 2 characters instead of looking at them individually (thanks to Ed Mpanduki for the inspiration!). This method can then be expanded to match any number of character strings as long as they conditions are all altered.
Ok, so how are we doing this? Well, firstly we need to define what the new evaluation is going to be – we are going to say we are looking for two characters, and that if together they equal \n then we are going to replace them with a new-line character. (We are also assuming you are dealing with a multi-line text field now as we are using new line formatting, so don’t forget to change yours if you’re just following on from the previous example)
If( IsMatch( Character1 & Character2, "([\\])([n])" ), Char(10), Character1)
We use Character1 & Character2 as this will combine them into a single string, and then when matching, I have created two separate groups with a single character inside each. Special characters like backslashes need to be ‘escaped’ by preceding them with another backslash as the Regex string uses a backslash as a functional character.
Now we need to Define how we are getting those two characters, So we need to cycle through them all sequentially and get the first and second character at the iteration point we are cycling through. We define the numeric Sequence to cycle through by using our previous Split formula that creates a table of character rows and wrapping it in the Sequence function which looks for a list of rows as its first parameter:
Sequence(CountRows(Split(TextInput1.Text,"")))
Then ForAll of the items in that sequence we are going to first define Character 1 and 2 using a With statement, then we are going to evaluate those characters using the IsMatch formula we created a moment ago. We Define Character one by taking the FirstN characters from our split string based on the current iteration of the ForAll which we can get from the Value property, and then taking the Last character from that (this cycles us through each character one by one based on the current iteration nubmer, ie if we are in iteration 2 then the first 2 items are pulled and we look at the last of those 2, which is the 2nd item).
For our second character, we check if the current iteration number is equal to the total number of characters from our split (in which case there can’t be a second character), and use Blank() if yes and if not then the same split as Character 1 but instead of Value, we use Value +1
We also need to consider the case where our formula has moved onto the next letter and looks back at the original first letter to ensure that it is comparing AND removing in two-letter chunks.
ForAll(
Sequence(CountRows(Split(TextInput1.Text,""))),
With(
{
Character1: Last(FirstN(Split(TextInput1.Text,""),Value)).Value,
Character2: If(Value = CountRows(Split(TextInput1.Text,"")),
Blank(),
Last(FirstN(Split(TextInput1.Text,""),Value +1)).Value
)
PreviousChar1: If(Value = CountRows(Split(TextInput1.Text,"")) || (Value=0),
Blank(),
Last(FirstN(Split(TextInput1.Text,""),Value -1)).Value)
},
{
CharacterEvaluation: If(IsMatch(Character1 & Character2, "([\\])([n])"), Char(10),
!(Value=0) && IsMatch(PreviousChar1 & Character1, "([\\])([n])"), Char(10),
Character1)
}
)
)
Now as the last step we Concat the resulting column as we did before and Set that to the variable we used for the timer in the previous example:
Set(gblSplitArrayNumbered,
Concat(
ForAll(
Sequence(CountRows(Split(TextInput1_1.Text,""))),
With(
{
Character1: Last(FirstN(Split(TextInput1.Text,""),Value)).Value,
Character2: If(Value = CountRows(Split(TextInput1.Text,"")),
Blank(),
Last(FirstN(Split(TextInput1.Text,""),Value +1)).Value
),
PreviousChar1: If(Value = CountRows(Split(TextInput1.Text,"")) || (Value=0),
Blank(),
Last(FirstN(Split(TextInput1.Text,""),Value -1)).Value)
},
{
CharacterEvaluation: If(IsMatch(Character1 & Character2, "([\\])([n])"), Char(10),
!(Value=0) && IsMatch(PreviousChar1 & Character1, "([\\])([n])"), Char(10),
Character1)
}
)
),
If(!(CharacterEvaluation = ""), CharacterEvaluation)
)
);
Reset(TextInput1_1);
(If we want to instead remove the two matching characters, we replace the Char(10) with "" and this will instead set those found instances to blank)
Conclusion
So, we’ve covered a few different ways to remove characters from strings using Regex in Power Apps, but we can also expand that further using additional logic or Regex to perform other functions. The options are endless!
I hope this has been helpful to you!
If you want to read my other Tips and Tricks, follow this link!
I constantly have folks copying and pasting from Excel…I need to get rid of all characters except digits ? i.e. I guess spaces carriage returns…etc..
Can Regex do that ?
Thanks great article
Hi David,
Absolutely, If you use the code in the “Change the text AS YOU TYPE” subheading, you can modify the Regex string to only allow through the numbers that were pasted using “([0-9])”, like this:
Set(gblTextRegex, Concat( Split(TextInput1.Text, “” ), If( IsMatch(Value, “([0-9])” ), Value))); Reset(TextInput1)
Cheers,
Sancho
Great article! When I put in the Concat( Split(Label1.Text, “” ), If( IsMatch(Result, “([A-Za-z0-9 ])” ), Result ) ), I got an error that Result is not defined
Hey Michael!
Microsoft stealth-updated the Split and Distinct functions to use Value instead of Result (I linked to the Power Apps version notes where they did that)
I have just updated the article with the newer formulae – let me know if any of them are still giving you trouble!
Cheers,
Sancho
I must be doing something really stupid because I can’t get past step 2. I create the two labels and when I try Split(Label1.Text, “”) I get the error “Expected Text Value”. I even tried replacing Label1.Text with an actual text string and get the same error. What am I doing wrong?
Hey Nathan,
As it says right above that – it creates a Table of records, not a text value, the next step is to reconstitute that table of values back into text using Concat 🙂
Cheers,
Sancho
Thank you for this post this is something I’ve been looking for. I was wondering is there a way to remove a combination of characters? If I have text like below, I want to remove ‘\n’, where I try Concat(Split(Label1.Text,””),If(IsMatch(Result,”([^\\n])”),Result)), it removes all the n’s as well
Lorem Ipsum is simply dummy text of the printing and typesetting industry.\n Lorem Ipsum has been the industry’s standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book
Hi,
Yes absolutely, I think if you prepare a variable that can be manipulated then you can separate that into groups of two by defining character 1 and 2 in a sequence.
So replace the timer code in the last step of the blog with the following:
I tested that with some Lorem and some other random added \n and it pulled them out of the string 🙂 You can of course modify that code to have it do something different if \n is found like insert a Char(10) new line
Thank you Sancho, this worked perfectly.
Great way of doing that! Thank you. I can’t tell if that last one allows hyphens or apostrophes, though. It’s important to allow those two, especially in a name field for those people who have hyphenated names or Irish names like O’Reilly.
Hi Valerie!
The formula there only covers capitals, lowercase, numbers and spaces. Normally we’d be using that kind of formula to ensure there are no special characters like hyphens or apostrophes so that when we have to pass that data to something that needs to create folders for example, then it will not fail due to the presence of special characters.
If you want to validate characters for names and include the hyphen and apostrophe characters you can simply add them to the regex string array (you can use the following Regex string in place of the one listed):
“[A-Za-z0-9 ‘-]”